Now that dotnet global tools are a thing, we are seeing an explosion of cool new cross platform command line apps being built with dotnet. A recent example of this is Giphy CLI, this is a tool to quickly get a gif url from giphy.com and markdown snippet ready for pasting.
My favourite terminal when using my Mac is iTerm2, it has lots of cool features, including the ability to display images right in the console! So I thought it would be great to add this feature to Giphy CLI, but first, how does it work?
Instructions from Docs
iTerm2 extends the xterm protocol with a set of proprietary escape sequences. In general, the pattern is:
ESC ] 1337 ; key = value ^G
Whitespace is shown here for ease of reading: in practice, no spaces should be used.
For file transfer and inline images, the code is:
ESC ] 1337 ; File = [optional arguments] : base-64 encoded file contents ^G
.NET Implementation
So to be honest, from mostly trial and error, I found that this is what you need to do from .NET:
where imageBytes
are the bytes of the image you want to display. You may also want to detect if you are running in iTerm in the first place, and only run this code if this is the case. Thankfully iTerm sets an environment variable we can use, named TERM_PROGRAM
, which will have the value iTerm.app
in the case where it's running under iTerm. Let's do that:
Here is the result:
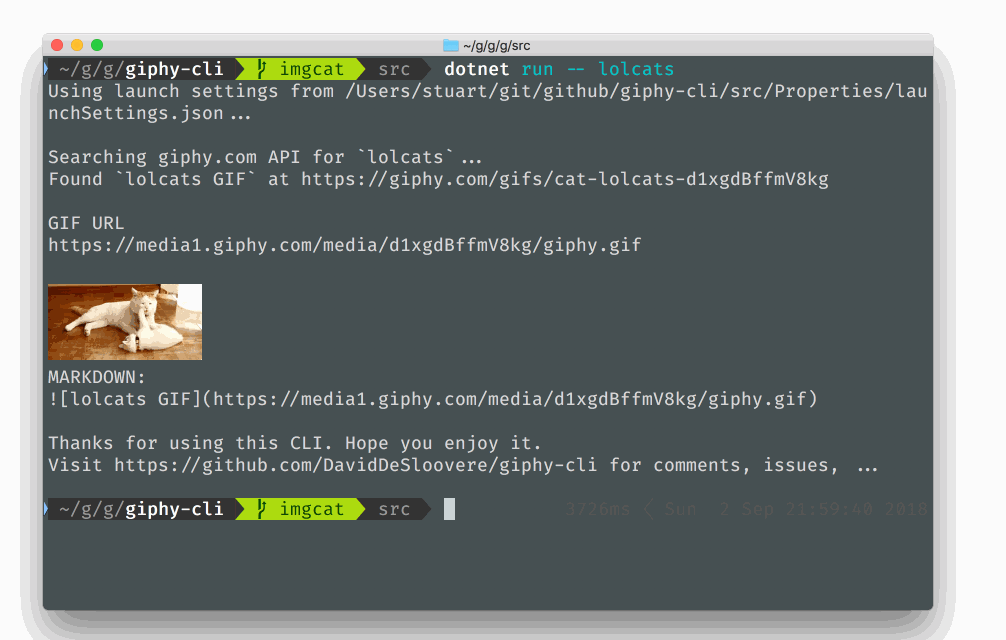
You can see this PR for a full working example:
Thanks to David De Sloovere for building this cool little tool.
Comments